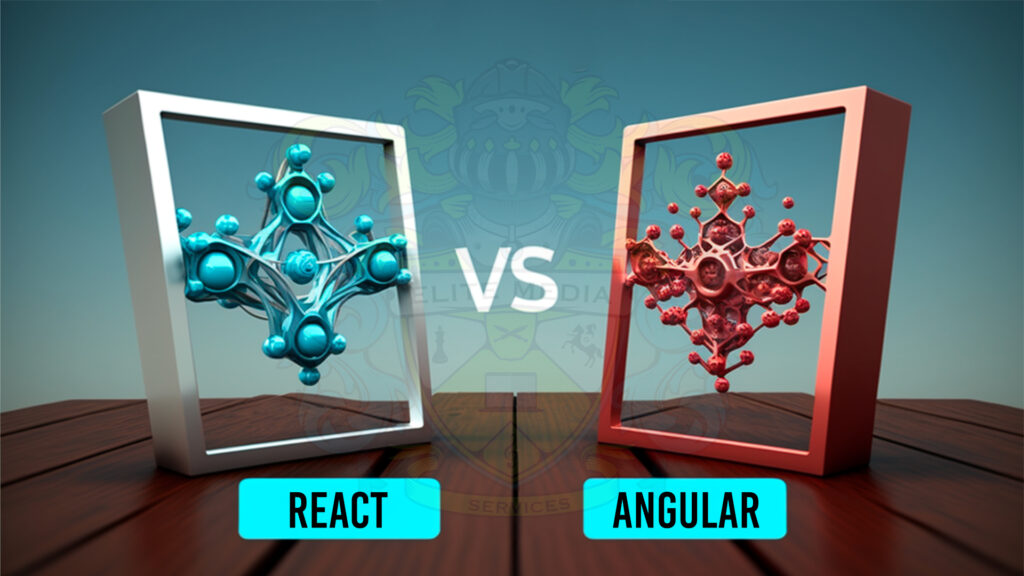
AngularJS is a front-end JavaScript framework for building web applications. It was developed by Google and provides a structure for building complex, large-scale web applications by breaking them down into smaller components.
Here are some examples to help illustrate how AngularJS works:
- Two-way data binding: AngularJS automatically keeps your model and view in sync, so when the model changes, the view updates, and vice versa. For example, if you change an input field in the view, the corresponding property in the model is updated automatically.
- Directives: AngularJS provides a set of built-in directives (such as
ng-show
,ng-repeat
, etc.) that can be used to add functionality to your HTML. For example, you can use theng-repeat
directive to loop over an array of items and display them in the view. - Modules: AngularJS allows you to organize your application into separate modules, each with its own set of controllers, services, and directives. This makes it easier to manage your application as it grows.
In comparison to ReactJS, AngularJS provides a more complete framework for building web applications. ReactJS, on the other hand, is focused solely on the view layer, and is often used in combination with other libraries such as Redux for state management. AngularJS also uses two-way data binding, while ReactJS uses one-way data flow.
Overall, the choice between AngularJS and ReactJS will depend on your specific needs and the complexity of your application. If you’re building a simple, single-page application, ReactJS might be a better choice, while if you’re building a more complex application, AngularJS might be a better fit.
Here’s an example of a simple counter component in ReactJS:
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
<button onClick={() => setCount(count - 1)}>Decrement</button>
</div>
);
};
export default Counter;
Here’s how the same component might look in AngularJS:
angular.module('myApp', [])
.controller('CounterController', function($scope) {
$scope.count = 0;
$scope.increment = function() {
$scope.count++;
};
$scope.decrement = function() {
$scope.count--;
};
})
.directive('counter', function() {
return {
restrict: 'E',
template: '<div>' +
'<p>Count: {{ count }}</p>' +
'<button ng-click="increment()">Increment</button>' +
'<button ng-click="decrement()">Decrement</button>' +
'</div>',
controller: 'CounterController'
};
});
As you can see, AngularJS uses a different approach to building components. Instead of using functional components and hooks like in ReactJS, AngularJS uses controllers and directives to define behavior and structure. AngularJS also uses two-way data binding, so updates to the model are automatically reflected in the view, and vice versa.